Well, you could do this:
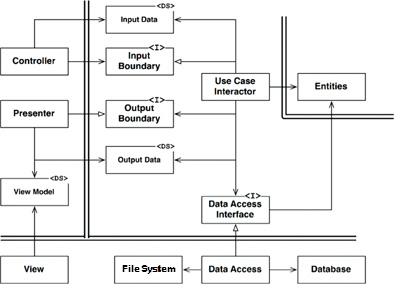
But if you do this:
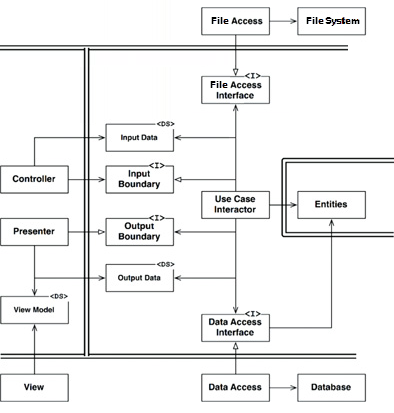
You have just as much flexibility and Clean Architecture DIP goodness and the Data Access stuff can focus on abstracting the DB.
I have started a flutter app and want to apply clean architecture to it. The first use case i have is the following:
I did a little reading about flutter. Didn't find any reason to care that you're using it in the context of this question other than it's very cross platform. So you'd benefit from having your core logic isolated from low level details. Which is something the buzzword architectures excel at.
"A user provides file(s)via "open file" dialogue.
That would be Input Data
coming in over an Input Boundary
. I assume this to be file names.
The provided files should be analyzed in first step.
Hold up. What files? Until they're open, the files might not exist regardless of what some asynchronous open file dialogue thinks. Files get deleted whenever the OS pleases. So don't just assume they still exist. Open them and see what happens. Doing that validates the file names.
After this, a summary with different statistics should be displayed. For example how many files were provided, whats the name of the files. Are the files valid. How many elements were found in the files etc."
Are some of the files missing? Is the directory missing?
Later on, the content in these files should be saved into database, but that would be the next use case.
As what? Blobs? Structured data that conforms to a schema? Not sure these are Entities yet.
My idea is to create a use case called "AnalyzeFilesUseCase" which returns a "AnalyzeReport" Entity in the end to the UI.
Not sure if these are being broken up as separate use cases so the user can stop at any point but ProcessFiles
that does all this stuff in on go might be a useful addition.
But here starts the trouble. Every implementation i found has a repository connected to the use case, where models are loaded from an identifier like ID first. But i get the data from the UI passed to the use case as input, so i don't have something from which i load it from the repository.
So? Not everything comes from the DB. Not everything ends up an entity. You are free to process the files into whatever data structures you wish.
Or should i pass the content from the provided files to the repository and return a entity out of it?
But if you make the files into Entities you need to be consistent with how you assign IDs.
Also: were do i put all the validation logic?
Validation should only happen when you know enough to validate properly. You should know the actual values of the data, what it's getting used for, and how to explain what's wrong with it. If you don't know all that here then here is a bad place to validate. Either find a better place or make here a better place.
That said, in Clean Architecture much of the validation happens in the Use Case Interactor
. But don't think it's the only place you can do it. Validate when the conditions are right for it.
Where to create the Report model etc? Is this everything in the use case class?
The Use Case Interactor
is a fine place to construct the Report model. Which sounds to me like Output Data
. Again, this can just be a data structure. Even if you plan to cram it into a DB focused entity later or even write it out as a file.
Some tipps for getting the right direction would be awesome
Understand, Clean Architecture isn't a method to solve this problem. It's a method to keep your solution flexible so you can solve the next problem more easily. Don't expect CA to make it fast or simple. Just flexible. The only good method I've found to check if you've achieved flexible code is to flex it.
Maybe I am wrong seeing the file content itself as user input transported via params into the use case.
That would put file access responsibilities on the controller rather than access files through an adapter.
Instead, the user just provides the file URIs and a file repository would load it and returns a "file" entity which is may be loaded from a file
That's what I'm showing in the second diagram.
.. but might be also loaded from anywhere else ...but with providing the URI nothing else than a file would make sense ... this is on the other hand again a sign of some lack in my approach ... hmmm ... i am confused –
Jim Panse
Once you can transform the file to a data structure that represents what your real needs you don't care if it came from a file. You care that you have the data structure you need. So long as that somehow gets created your needs are met.
Now sure, if you want to let people recite data into a microphone to populate that data structure you're going to need different code to transform it. But once transformed into a common data structure you don't care if it came from a file, DB, or a spoken word poem.
The wrinkle comes when it's time to put it back out. Just as transformation might be required coming in it can be required going out. Many designs ignore this and let external details (DB, file, etc...) creep into their data structures and entities. Which seems to work fine, so long as they only ever use that one medium.
I've worked in places where we didn't just have one. We ended up creating our own internal data structures and entities. Ones that focused on our needs. We wrote ways to transform the internals to externals. Thus we ended up with different kinds of Entities focused on different details.
That works, it's not the purist ideal, but it works.
You were asking about giving the file info an ID. That's where you cross the line from value object to entity. That's not a Clean Architecture issue. To understand that better I suggest studying Domain Driving Design.